ES6 Arrow Function
ES6 is an in-depth series which has many features, that makes the life of a developer much easier and one of them is the arrow function. If you don’t know about ES6, I have a brief introduction article on it, have a read.
An arrow function is one of the most important topics in the land of ES6, if you ever watched any tutorial of ES6 before, you would have surely heard of arrow functions, or if not ES6 specifically if you have watched tutorials for some javascript libraries/frameworks you might have seen the arrow function.
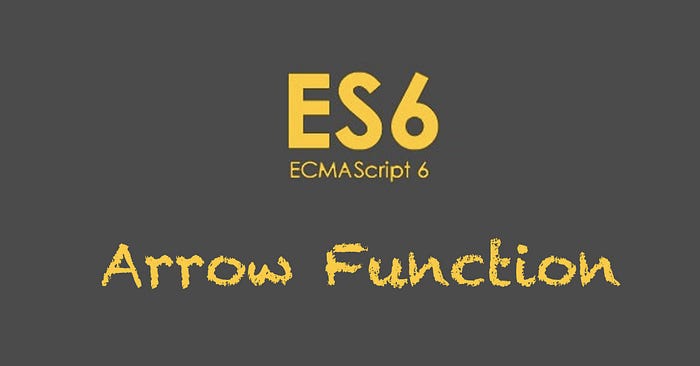
There is nothing wrong with traditional ES5 function if you like to use them, you can continue using the old ES5 function but you should learn it because if you are into a project i.e. not from scratch and another developer has already written some of his code and he/she has used arrow functions but you don’t know about it, that will blow your mind.
Let’s see below an example for ES6 function, we will use a fat arrow in ES6 functions, writing =(equal sign) and >(greater than sign) together gives => this is a fat arrow.
In simple words, in ES6 they just removed the function keyword from old syntax and put a => after parameters and formed arrow function but there are other improvements as well but in terms of syntax this is it.
//Let's first see ES5 functionconst add1 = function(a,b){
return a + b;
}
add1(1,2); //Output: 3//In ES6 arrow function they just removed the function keywordconst add2 = (a,b) => {
return a+b;
}
add2(1,2); //Output: 3//If your function has a single javascript expression you can remove the return keyword and curly braces//One way
const add3 = (a,b) => {
a+b;
}
add3(1,2); //Output: 3//One more way
const add4 = (a,b) => a + b;
add4(1,2); //Output: 3
Yes, you saw it right. An arrow function is just a one-line function if your function has only one expression. Simple isn’t it?
//ES6 arrow function to square a numberconst square = (a) => a*a;
square(9) //Output: 81//If your function has a single javascript expression and a single parameter you can remove the curly braces and return keyword as well as parenthesis around parameterconst square = a => a*a;
square(9) //Output: 81
Arrow functions are pretty good for reducing the number of lines in your code but annoying sometimes if you don’t strictly follow the rules, So keep practising it and keep these rules in your mind.
//Let’s use arrow function with array helper methodconst nums = [1,2,3,4,5,6];
let sqrNums = [];//ES5 function and map array helper to square numbers of array
sqrNums = nums.map(function(num){
return num*num;
});//ES6 arrow function and map array helper to square numbers of array
sqrNums = nums.map(num => num*num);
Except this compact syntax, there is one more benefit of ES6 arrow function i.e. really very important, you would have used this keyword many times and in javascript, you know how annoying it is, this in javascript never refers to what you want it to refer to but ES6 solves the problem for you.
Let’s see what problem occurs with this keyword in ES5
Let us take an example of an object named team having two players, a team name and teamInfo function prints name of players with team name.
Expecting Output an array [“Kohli is in Super Sixers team.”,”Huss is in Super Sixers team.”,”Clarke is in Super Sixers team.”,”Watto is in Super Sixers team.”,”Steyn is in Super Sixers team.”]
const team = {
players:[“Kohli”,”Huss”,”Clarke”,”Watto”,”Steyn”],
teamName: “Super Sixers”,
teamInfo: function(){
return this.players.map(function(player){
return `${player} is in ${this.teamName} team.`;
});
}
}
team.teamInfo(); //Error
This will cause TypeError: Cannot read property ‘teamName’ of undefined because this here not referring to object team instead the value of this coming is undefined. To solve this problem we have two options.
- use bind(this) i.e. bind the function’s context to the current context.
const team = {
players:[“Kohli”,”Huss”,”Clarke”,”Watto”,”Steyn”],
teamName: “Super Sixers”,
teamInfo: function(){
return this.players.map(function(player){
return `${player} is in ${this.teamName} team.`;
}.bind(this));
}
}
team.teamInfo();
2. by creating a variable self and assigning the value of this to it.
const team = {
players:[“Kohli”,”Huss”,”Clarke”,”Watto”,”Steyn”],
teamName: “Super Sixers”,
teamInfo: function(){
let self=this;
return this.players.map(function(player){
return `${player} is in ${self.teamName} team.`;
});
}
}
team.teamInfo();
Now see how ES6 arrow function solves the problem.
By using arrow function inside the map method and this
will work well with this little tiny change.
const team = {
players:[“Kohli”,”Huss”,”Clarke”,”Watto”,”Steyn”],
teamName: “Super Sixers”,
teamInfo: function(){
return this.players.map(player => {
return `${player} is in ${this.teamName} team.`;
});
}
}
team.teamInfo();
You might be wondering what actually happened what made this work perfectly, the answer is fat arrow function automatically binds the value for all the code inside the function, the fat arrow function makes the value of this behave as we expect it to. To know more about the lexical environment. Click the link.
I have tried to explain these arrow function in a very short and simple way. I will try to write more articles explaining all the syntaxes of ES6. Hope it was useful.
Reading is good but reading with implementation is great!
Suggestions and critics about the article are most welcome.